You need to sign in to do that
Don't have an account?

74% code coverage of test class
Hello,
I have written a test class. Its code coverage is 745. I want to increase its coverage.
Test class:
@isTest
private class CreateNewQuoteTest {
private static testMethod void testManipulateOrders(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id ;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Opp';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
update o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
o.StageName = 'Closed Won';
o.Invoice__r.size();
system.debug('test---------o.StageName'+o.StageName);
system.debug('test---------o.Invoice__r.size()'+o.Invoice__r.size());
if(o.StageName == 'Closed Won' && o.Invoice__r.size() == 0){
Invoice__c i2 = new Invoice__c();
system.debug('test---------Invoice'+i2);
}
update o;
test.StopTest();
}
private static testMethod void testManipulateOrders1(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Test opportunity';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
update o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
o.StageName = 'Closed Won';
o.Delivery_Notes__r.size();
system.debug('test---------o.StageName'+o.StageName);
system.debug('test---------o.Delivery_Notes__r.size()'+o.Delivery_Notes__r.size());
if(o.StageName == 'Closed Won' && o.Delivery_Notes__r.size() == 0)
{
Delivery_Notes__c newDelivery = new Delivery_Notes__c();
system.debug('test---------Delivery'+newDelivery);
}
//update o;
else if(o.StageName != 'Closed Won' && o.Delivery_Notes__r.size() != 0)
{
system.debug('o.StageName == Closed Won && o.Delivery_Notes__r.size() == 0');
}
test.StopTest();
}
private static testMethod void testManipulateOrders2(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Test opportunity';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Order od = new Order();
od.Name = 'Test';
od.AccountId = a.Id;
od.status = 'Draft';
od.Your_Order_Reference__c = '123';
od.EffectiveDate = system.today();
od.Pricebook2Id =customPB.Id;
od.OpportunityId = o.Id;
od.QuoteId= q.id;
insert od;
// update od;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
od.status = 'Closed Won';
od.Orders.size();
system.debug('test--------- od.status'+ od.status);
system.debug('test---------od.Orders.size()'+od.Orders.size());
if( od.status == 'Closed Won' && od.Orders.size() == 0)
{
Order newOrder = new Order();
newOrder.AccountId = a.Id;
newOrder.OpportunityId = o.Id;
// newOrder.QuoteId = q.SyncedQuoteId;
newOrder.Status = 'Closed Won';
newOrder.EffectiveDate = system.today();
newOrder.Pricebook2Id = o.Pricebook2Id;
insert newOrder;
system.debug('test---------Order'+newOrder);
}
update od;
test.StopTest();
}
}
Trigger:
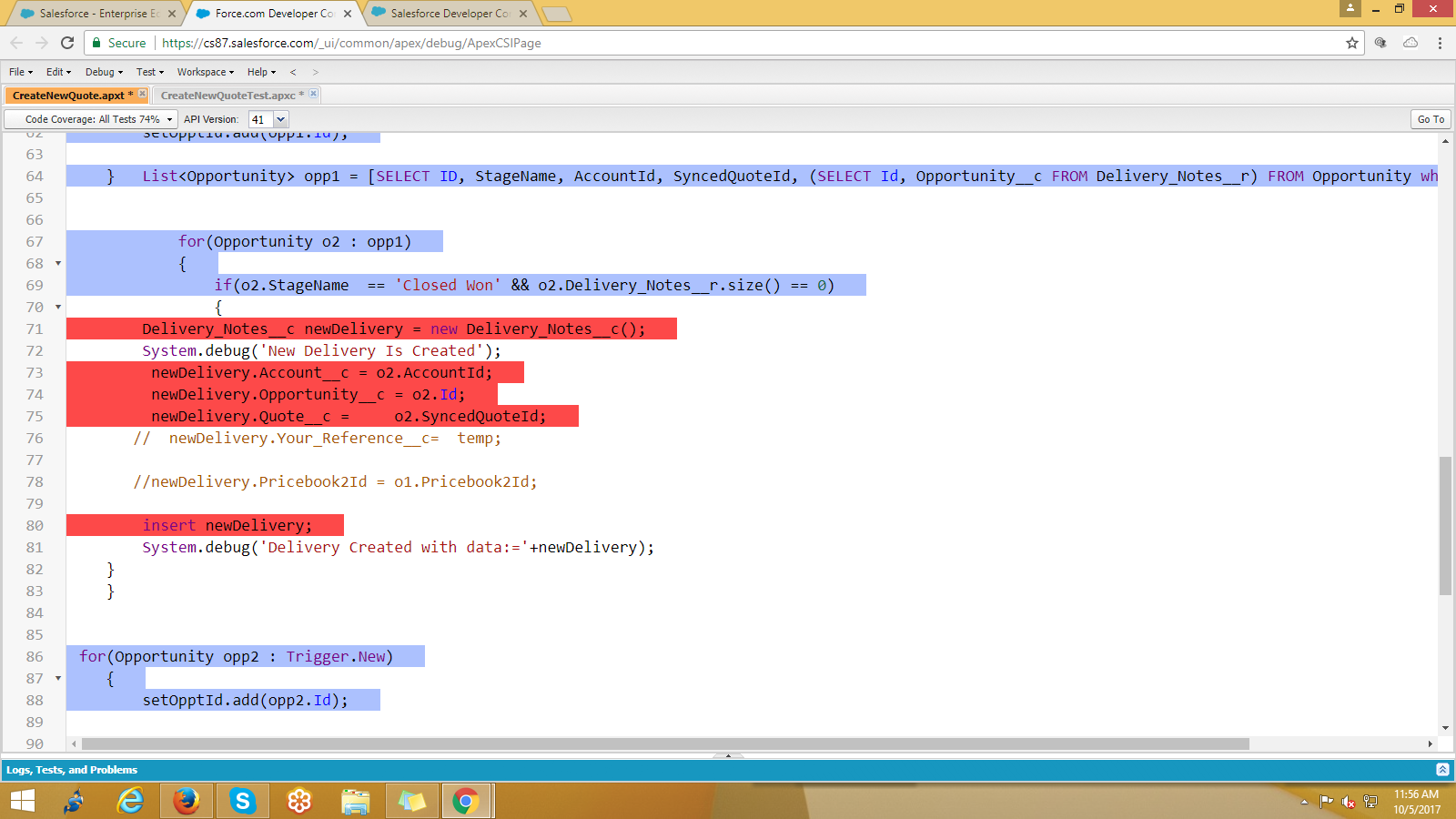
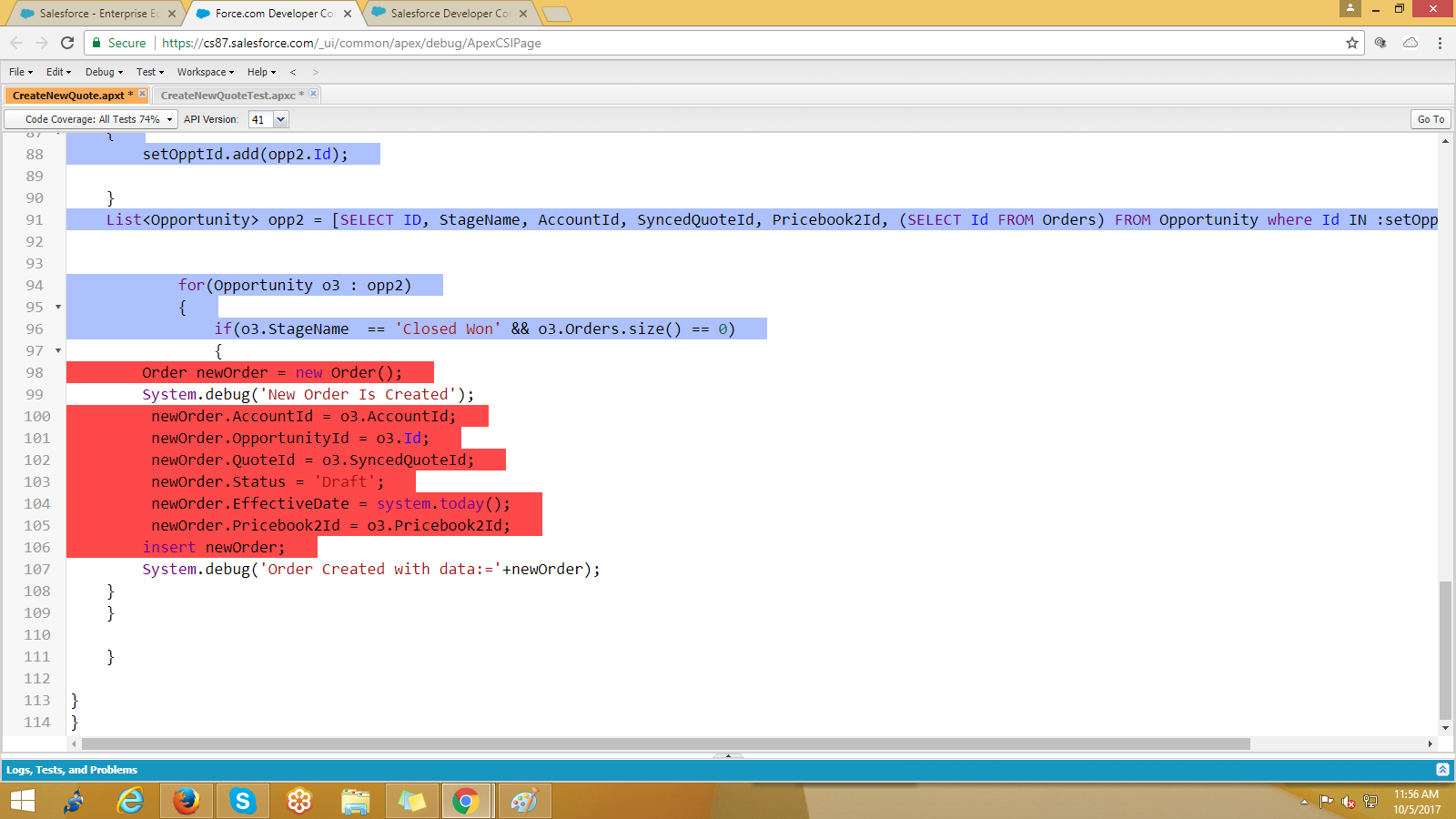
Its giving an error System.DmlException: Update failed. First exception on row 0 with id 0068E00000BqCLfQAN; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, CreateNewQuote: execution of AfterUpdate
caused by: System.DmlException: Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, CreateInvoiceLineItem: execution of AfterInsert
caused by: System.QueryException: List has no rows for assignment to SObject
Thanks & regards,
Utz
I have written a test class. Its code coverage is 745. I want to increase its coverage.
Test class:
@isTest
private class CreateNewQuoteTest {
private static testMethod void testManipulateOrders(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id ;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Opp';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
update o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
o.StageName = 'Closed Won';
o.Invoice__r.size();
system.debug('test---------o.StageName'+o.StageName);
system.debug('test---------o.Invoice__r.size()'+o.Invoice__r.size());
if(o.StageName == 'Closed Won' && o.Invoice__r.size() == 0){
Invoice__c i2 = new Invoice__c();
system.debug('test---------Invoice'+i2);
}
update o;
test.StopTest();
}
private static testMethod void testManipulateOrders1(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Test opportunity';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
update o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
o.StageName = 'Closed Won';
o.Delivery_Notes__r.size();
system.debug('test---------o.StageName'+o.StageName);
system.debug('test---------o.Delivery_Notes__r.size()'+o.Delivery_Notes__r.size());
if(o.StageName == 'Closed Won' && o.Delivery_Notes__r.size() == 0)
{
Delivery_Notes__c newDelivery = new Delivery_Notes__c();
system.debug('test---------Delivery'+newDelivery);
}
//update o;
else if(o.StageName != 'Closed Won' && o.Delivery_Notes__r.size() != 0)
{
system.debug('o.StageName == Closed Won && o.Delivery_Notes__r.size() == 0');
}
test.StopTest();
}
private static testMethod void testManipulateOrders2(){
Pricebook2 customPB = new Pricebook2(Name='SDEC GBP Distributor Price Sheet', isActive=true);
insert customPB;
Account a = new Account();
a.Name = 'Test Account';
a.Price_Book__c = customPB.id;
insert a;
Contact c = new Contact();
c.LastName='test';
insert c;
Product2 p = new Product2();
p.Name = 'Test Product ';
p.Description='Test Product Entry 1';
p.productCode = 'ABC';
p.isActive = true;
p.Family = 'Blackbox';
insert p;
Id pricebookId = Test.getStandardPricebookId();
system.debug('standardPb--------------->test'+pricebookId);
PricebookEntry standardPrice = new PricebookEntry();
standardPrice.Pricebook2Id = pricebookId;
standardPrice.Product2Id = p.Id;
standardPrice.UnitPrice = 1;
standardPrice.IsActive = true;
standardPrice.UseStandardPrice = false;
insert standardPrice ;
PricebookEntry custompbe2 = new PricebookEntry();
custompbe2.Pricebook2Id = customPB.Id;
custompbe2.Product2Id = p.Id;
custompbe2.UnitPrice = 1;
custompbe2.IsActive = true;
custompbe2.UseStandardPrice = false;
insert custompbe2 ;
Opportunity o = new Opportunity();
o.Name = 'Test opportunity';
o.AccountId = a.Id;
o.CloseDate= system.today();
o.StageName = 'Proposal';
insert o;
Quote q = new Quote();
q.Name= o.Id;
q.OpportunityId = o.Id;
q.status = 'Draft';
q.Pricebook2Id = customPB.id;
insert q;
Order od = new Order();
od.Name = 'Test';
od.AccountId = a.Id;
od.status = 'Draft';
od.Your_Order_Reference__c = '123';
od.EffectiveDate = system.today();
od.Pricebook2Id =customPB.Id;
od.OpportunityId = o.Id;
od.QuoteId= q.id;
insert od;
// update od;
Invoice__c i = new Invoice__c();
i.Account__c = a.Id;
i.Quote__c = q.Id;
insert i;
Delivery_Notes__c d = new Delivery_Notes__c();
d.Account__c = a.id;
d.Opportunity__c = o.Id;
d.Quote__c = q.Id;
insert d;
test.StartTest();
od.status = 'Closed Won';
od.Orders.size();
system.debug('test--------- od.status'+ od.status);
system.debug('test---------od.Orders.size()'+od.Orders.size());
if( od.status == 'Closed Won' && od.Orders.size() == 0)
{
Order newOrder = new Order();
newOrder.AccountId = a.Id;
newOrder.OpportunityId = o.Id;
// newOrder.QuoteId = q.SyncedQuoteId;
newOrder.Status = 'Closed Won';
newOrder.EffectiveDate = system.today();
newOrder.Pricebook2Id = o.Pricebook2Id;
insert newOrder;
system.debug('test---------Order'+newOrder);
}
update od;
test.StopTest();
}
}
Trigger:
Its giving an error System.DmlException: Update failed. First exception on row 0 with id 0068E00000BqCLfQAN; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, CreateNewQuote: execution of AfterUpdate
caused by: System.DmlException: Insert failed. First exception on row 0; first error: CANNOT_INSERT_UPDATE_ACTIVATE_ENTITY, CreateInvoiceLineItem: execution of AfterInsert
caused by: System.QueryException: List has no rows for assignment to SObject
Thanks & regards,
Utz
Check your code, there will be some query had been written, which return zero rows, thats why you got this error:
"caused by: System.QueryException: List has no rows for assignment to SObject"
Check what are the query you have written in your code. After the test class run completes, check the query have return values or not.
If it helps you, please mark is as best answer, so it will be helpful for other developers.