You need to sign in to do that
Don't have an account?

VF page with Apex logic
Hi all! This is my first post on this community forum, I am an absolute beginner and currently studying Salesforce development by my own.
I am trying to create a Visualforce page with Positions and the ability to filter by Position status ( Opened, Closed). The Status field should be editable and have a Save button.
Like this:
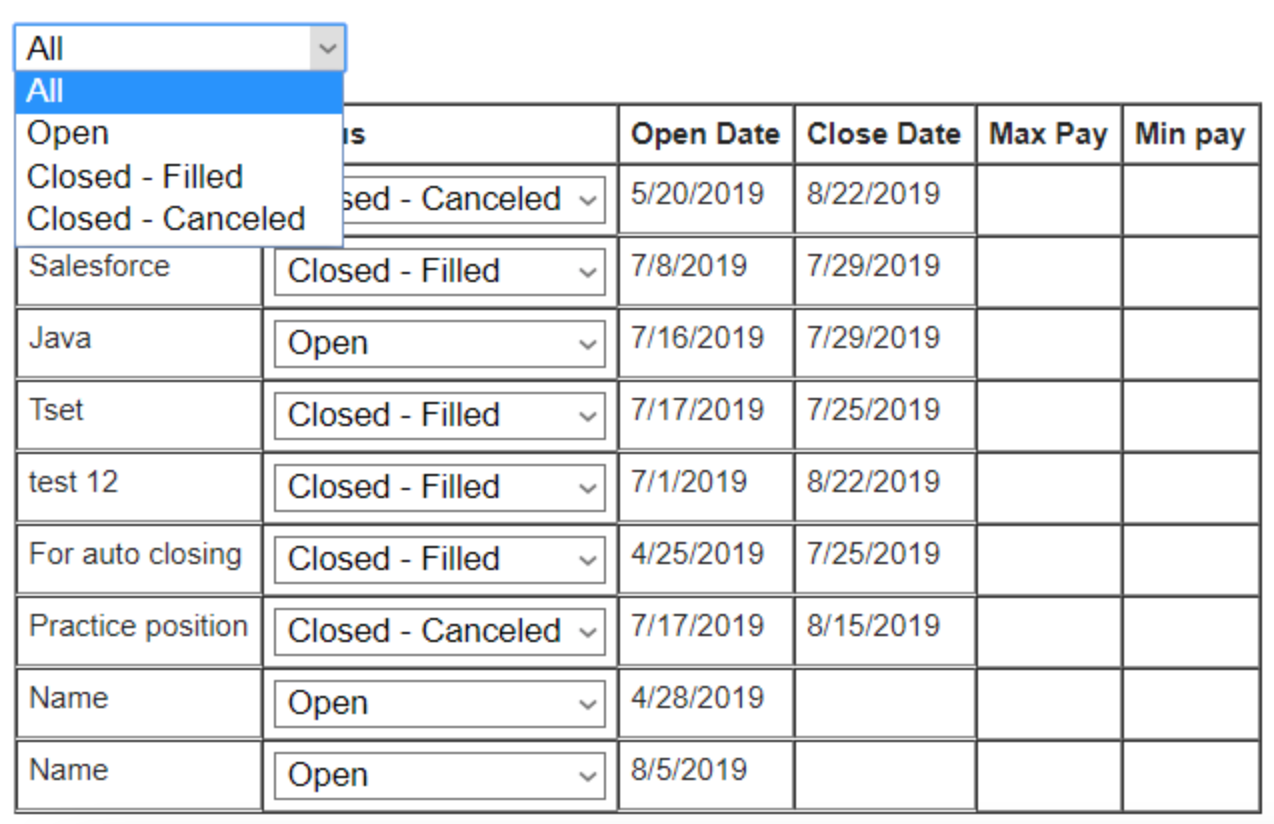
This is my code VF:
<apex:page controller="PositionCustomController" showHeader="true" sidebar="true">
<apex:form>
<apex:pageBlock title="Positions list">
<apex:pageBlockTable value="{!positions}" var="pos">
<apex:inputField value="{!pos.Status__c}">
<apex:commandButton action="{! save}" value="Save"></apex:commandButton>
</apex:inputField>
<apex:column value="{!pos.Name}"></apex:column>
<apex:column value="{!pos.Status__c}"></apex:column>
<apex:column value="{!pos.Opening_date__c}"></apex:column>
<apex:column value="{!pos.Closing_date__c}"></apex:column>
<apex:column value="{!pos.Salary_max__c}"></apex:column>
<apex:column value="{!pos.Salary_min__c}"></apex:column>
</apex:pageBlockTable>
</apex:pageBlock>
</apex:form>
</apex:page>
Apex:
public with sharing class PositionCustomController{
public List<Position__c> getPositions(){
List<Position__c> results = Database.query(
'SELECT Name,Status__c,Opening_date__c,Closing_date__c,Salary_max__c,Salary_min__c from Position__c' );
return results;
}
}
I know this code is shitty, but I am struggling and need help, it is super discouraging at the moment. Thanks!
I am trying to create a Visualforce page with Positions and the ability to filter by Position status ( Opened, Closed). The Status field should be editable and have a Save button.
Like this:
This is my code VF:
<apex:page controller="PositionCustomController" showHeader="true" sidebar="true">
<apex:form>
<apex:pageBlock title="Positions list">
<apex:pageBlockTable value="{!positions}" var="pos">
<apex:inputField value="{!pos.Status__c}">
<apex:commandButton action="{! save}" value="Save"></apex:commandButton>
</apex:inputField>
<apex:column value="{!pos.Name}"></apex:column>
<apex:column value="{!pos.Status__c}"></apex:column>
<apex:column value="{!pos.Opening_date__c}"></apex:column>
<apex:column value="{!pos.Closing_date__c}"></apex:column>
<apex:column value="{!pos.Salary_max__c}"></apex:column>
<apex:column value="{!pos.Salary_min__c}"></apex:column>
</apex:pageBlockTable>
</apex:pageBlock>
</apex:form>
</apex:page>
Apex:
public with sharing class PositionCustomController{
public List<Position__c> getPositions(){
List<Position__c> results = Database.query(
'SELECT Name,Status__c,Opening_date__c,Closing_date__c,Salary_max__c,Salary_min__c from Position__c' );
return results;
}
}
I know this code is shitty, but I am struggling and need help, it is super discouraging at the moment. Thanks!
I have built/implemented similar visualforce page in my dev org with Case object, you can try to use something like below:
My VF page: Apex: The UI looks as expected by you:
Please modify the same visualforce to fit with your custom object and it should work for you too..
Please mark as Best Answer so that it can help others in the future.
Thanks.
All Answers
I have built/implemented similar visualforce page in my dev org with Case object, you can try to use something like below:
My VF page: Apex: The UI looks as expected by you:
Please modify the same visualforce to fit with your custom object and it should work for you too..
Please mark as Best Answer so that it can help others in the future.
Thanks.