You need to sign in to do that
Don't have an account?

How can I get more coverage of my code with these test classes?
It is the first time that I write test classes and it is being a bit complicated. With these test classes I am only getting coverage for the beginning part of the batch.
Class 1:
Class 2:
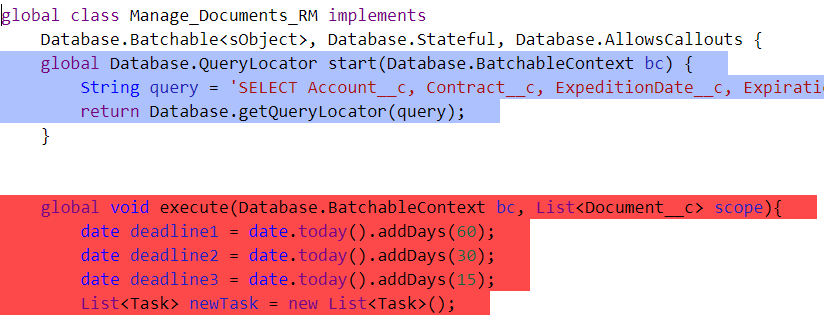
Class 1:
global class Manage_Documents_RM implements Database.Batchable<sObject>, Database.Stateful, Database.AllowsCallouts { global Database.QueryLocator start(Database.BatchableContext bc) { String query = 'SELECT Account__c, Contract__c, ExpeditionDate__c, ExpirationDate__c, Contact__c, OwnerId, Id FROM Document__c WHERE ExpirationDate__c > LAST_N_DAYS:60 OR ExpirationDate__c < TODAY'; return Database.getQueryLocator(query); } global void execute(Database.BatchableContext bc, List<Document__c> scope){ date deadline1 = date.today().addDays(60); date deadline2 = date.today().addDays(30); date deadline3 = date.today().addDays(15); List<Task> newTask = new List<Task>(); Set<String> myIds = new Set<String>(); Task[] cList = [Select ID, Subject, WhatId FROM Task WHERE Subject = 'Update Due Date' AND Status = 'Open']; Set<String> exstIds = new Set<String>(); for(Task c : cList) { exstIds.add(c.WhatId); } for (Document__c document : scope) { if(document.ExpirationDate__c <= deadline1 || document.ExpirationDate__c <= deadline2 || document.ExpirationDate__c <= deadline3) { Task tsk = new Task(); tsk.RecordTypeId = SObjectType.Task.getRecordTypeInfosByDeveloperName().get('CallReport').getRecordTypeId(); tsk.WhatId = document.Id; tsk.Subject = 'Update Due Date'; tsk.Status = 'Open'; tsk.Priority = 'Normal'; tsk.OwnerId = document.OwnerId; tsk.ActivityDate = document.ExpirationDate__c; if(exstIds.isEmpty() || !exstIds.Contains(document.Id)) { newTask.add(tsk); } } } insert newTask; } global void finish(Database.BatchableContext bc){ } }Test Class 1:
@isTest public class Manage_Documents_RM_Test { static testmethod void ManageDocumentsRMTestMethod(){ Task tsk = new Task(Subject='Teste', Status='Open'); insert tsk; Manage_Documents_RM a = new Manage_Documents_RM(); Database.executeBatch(a); } }
Class 2:
global class SendEmailToOwnerTask implements Database.Batchable<sObject> { Map<string,List<Task>> userEmailTasklistmap = new Map<string,List<Task>>(); global Database.QueryLocator start(Database.BatchableContext BC){ return Database.getQueryLocator([SELECT ID, CreatedDate, What.Id, What.Name, Owner.Email, OwnerId, Owner.Name, Status, ActivityDate, Subject from Task WHERE Status != 'Completed' and Owner.IsActive = true]); } global void execute(Database.BatchableContext BC, List<Task> scope){ for(Task Tsk : scope){ if(!userEmailTasklistmap.Containskey(tsk.Owner.Email)){ userEmailTasklistmap.put(tsk.Owner.Email, new List<Task>()); } userEmailTasklistmap.get(tsk.Owner.Email).add(tsk); } List<Messaging.SingleEmailMessage> mails = new List<Messaging.SingleEmailMessage>(); for(string email : userEmailTasklistmap.keyset()){ Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage(); list<string> toAddresses = new list<string>(); toAddresses.add(email); mail.setToAddresses(toAddresses); mail.setSubject('Tarefas em aberto'); String username = userEmailTasklistmap.get(email)[0].Owner.Name; String htmlBody = ''; htmlBody = '<body style="height: auto; min-height: auto; font-family: Arial, sans-serif; font-style: normal; font-weight: normal; font-size: 14px; line-height: 16px;">'+ +'<style type="text/css">p {margin: 0 0 15px;}</style>'+ +'<div style="max-width: 550px; width: 100%; margin: 0 auto;">'+ +'<div style="background: #001489; padding: 50px 0; text-align: center;"><img alt="ab" src="logo.png" style="width: 200px; height: 60px; display: inline-block;" title="AB" /></div>'+ +'<div style="padding: 45px 35px; background: #fff;">'+ +'<p style="font-weight: bold; font-size: 24px; line-height: 28px; margin-bottom: 15px;">Tarefas em aberto</p></div>'+ +'Hey, ' + username + '! Check these open Tasks.<br/><br/>'; for(Task tsk : userEmailTasklistmap.get(email)){ String duedate = ''; if (tsk.ActivityDate != null) duedate = tsk.ActivityDate.format(); else duedate = ''; String Subject = tsk.subject; String wn = tsk.what.name; string what = tsk.what.id; string link = URL.getSalesforceBaseUrl().toExternalForm()+'/'+ tsk.id; htmlBody += 'Subject: ' + Subject + '<br/>Document: ' + wn + '<br/>Due Date: ' + duedate + '<br/>Link: ' + link + '<br/><br/>'; } String label = Label.Mensagem_MarginEmail; htmlBody += '<div style="border-top: 9px solid #001489; background: #fff; padding: 15px 35px;">'+ +'<p style="font-size: 9px; text-align: center; line-height: 10px; color: #5B5B5B; margin: 0;">'+ label +'</p></div>'+ +'<div style="padding: 25px 0;">'+ +'<p style="font-size: 9px; line-height: 10px; text-align: center; color: #141C4B;">De: <a href="" style="font-size: 9px; line-height: 10px; text-align: center; color: #141C4B; text-decoration: underline;">Salesforce</a></p>'+ +'</div></div></body>'; mail.sethtmlBody(htmlBody); mails.add(mail); } if(mails.size()>0) Messaging.sendEmail(mails); } global void finish(Database.BatchableContext BC){ } }
Please follow below code:-
if you need any assistanse, Please let me know!!
Kindly mark my solution as the best answer if it helps you.
Thanks
Mukesh
All Answers
Since this code requires an understanding of your implementation, it might not be possible to provide exact edit suggestions. However, the below articles give a good insight into how coverage can be improved
https://salesforce.stackexchange.com/questions/244794/how-do-i-increase-my-code-coverage-or-why-cant-i-cover-these-lines
Examples of code coverage of execute method in test classes:
https://salesforce.stackexchange.com/questions/62/unit-testing-code-which-has-logic-around-the-createddate https://salesforce.stackexchange.com/questions/202421/execute-method-on-batch-returns-0-code-coverage
If this information helps, please mark the answer as best. Thank you
Please follow below code:-
if you need any assistanse, Please let me know!!
Kindly mark my solution as the best answer if it helps you.
Thanks
Mukesh
User us = [Select id from User where Id = :UserInfo.getUserId()];
Account a = new Account();
a.Name = 'Testing Class';
a.OwnerId = us.Id;
Document__c doc = new Document__c();
doc.Name = 'Test Document';
doc.ExpirationDate__c = Date.newInstance(2021, 5, 31);
doc.OwnerId = a.OwnerId;
Insert doc;
System.runAs(us) {
Test.StartTest();
Manage_Documents_RM instancevar = new Manage_Documents_RM();
ID batchprocessid = Database.executeBatch(instancevar);
Test.StopTest();
}