You need to sign in to do that
Don't have an account?

create a VF page to display Account Parent,child and grandchild names
create a VF page to display Account Parent,child and grandchild names like the screenshot below.Please help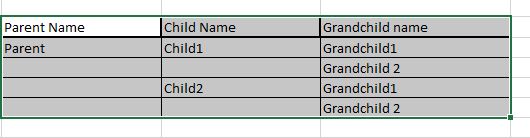
function readOnly(count){ }
You need to sign in to do that
Don't have an account?
You can use the below code:
<<<<<<-----VF Page ------>>>>>
<<<<<<<<<<------Controller---->>>>>>>>>
I hope you find the above solution helpful. If it does, please mark as Best Answer to help others too.
Thanks,
Ajay Dubedi
All Answers
Greetings to you!
Please refer to the below links which might help you further with the above requirement.
http://www.infallibletechie.com/2013/11/how-to-display-parent-child-and.html
http://salesforceblogger.blogspot.com/2012/03/hierarchy-in-visualforce.html
I hope it helps you.
Kindly let me know if it helps you and close your query by marking it as solved so that it can help others in the future. It will help to keep this community clean.
Thanks and Regards,
Khan Anas
You can use the below code:
<<<<<<-----VF Page ------>>>>>
<<<<<<<<<<------Controller---->>>>>>>>>
I hope you find the above solution helpful. If it does, please mark as Best Answer to help others too.
Thanks,
Ajay Dubedi