You need to sign in to do that
Don't have an account?

how to get all the fields of an selected object(standard and custom) using lightning component
i had populate all the objects name in the org by using "iteration" then my requirement is whenever i onclick any object i need to display all fields related to that object pls anyone help me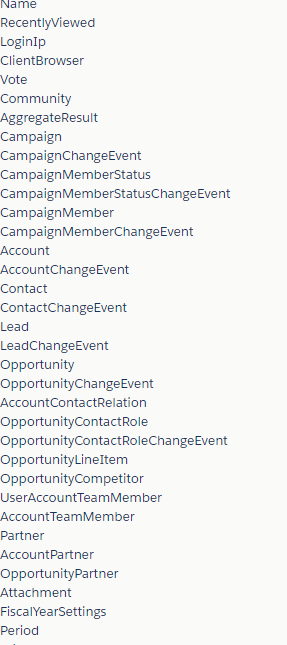
I suggest that you create a visualforce page with a dynamic picklist of sobjects, and a custom button with an apex method that returns all the available field names for that object.
http://www.salesforce.com/us/developer/docs/api/Content/sforce_api_calls_describesobject.htm
For your reference, you can check the below blog,
https://developer.salesforce.com/forums/?id=9060G000000XbuqQAC
I hope you find the above information is helpful. If it does, please mark as Best Answer to help others too.
Thanks.
I hope you find this helpful. If it does, please mark as Best Answer to help others too.
Thanks.
Pankaj :-)