You need to sign in to do that
Don't have an account?

Lightning Web Component not showing API call results
Hello!
I'm working with a pretty basic Lightning Web Component. I've followed this guide loosely. https://trailhead.salesforce.com/en/content/learn/projects/lwc-build-flexible-apps/record-list
The only differentiation is that I'm using a different API.
Here is my js file
I can run it in anonymous window and see results. I also see the aura log that proves it is being called. It returns 10 records, but when I view the results the objects returned are just blank objects.
I'm not sure why it is showing up as blank. Am I being hit by some kind of limit when data is being sent to the front end?
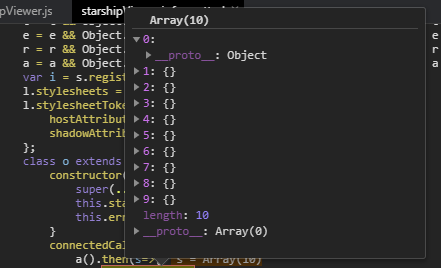
I'm working with a pretty basic Lightning Web Component. I've followed this guide loosely. https://trailhead.salesforce.com/en/content/learn/projects/lwc-build-flexible-apps/record-list
The only differentiation is that I'm using a different API.
Here is my js file
import { LightningElement, wire } from 'lwc'; import getStarships from '@salesforce/apex/StarshipController.getStarships'; export default class StarshipViewer extends LightningElement { starships; error; connectedCallback(){ getStarships() .then(result => { this.starships = result; }) .catch(error => { this.error = error; }); } }Here is my controller
public inherited sharing class StarshipController { @AuraEnabled public static List<Starship> getStarships(){ List<Starship> starships = new List<Starship>(); string strEndPointURL = 'https://swapi.dev/api/starships/'; starships = getStarships(strEndPointURL); system.debug(starships.size()); return starships; } private static List<Starship> getStarships(string strEndPointURL){ String strResponse = null; if(String.isNotBlank(strEndPointURL)) { HttpRequest request = new HttpRequest(); request.setEndpoint(strEndPointURL); request.setMethod('GET'); request.setHeader('Accept', 'application/json'); try { Http http = new Http(); HttpResponse response = http.send(request); if (response.getStatusCode() == 200 ) { strResponse = response.getBody(); } else { throw new CalloutException(response.getBody()); } } catch(Exception ex) { throw ex; } } if(!String.isBlank(strResponse)) { JSON2Apex res = JSON2Apex.parse(strResponse); return res.results; } else { return new List<Starship>(); } } }
I can run it in anonymous window and see results. I also see the aura log that proves it is being called. It returns 10 records, but when I view the results the objects returned are just blank objects.
I'm not sure why it is showing up as blank. Am I being hit by some kind of limit when data is being sent to the front end?
All Answers
@AuraEnabled (cacheable=true)
public with sharing class JSON2Apex {
public Integer count {get;set;}
public String next {get;set;}
public Object previous {get;set;}
public List<Starship> results {get;set;}
public JSON2Apex(JSONParser parser) {
while (parser.nextToken() != System.JSONToken.END_OBJECT) {
if (parser.getCurrentToken() == System.JSONToken.FIELD_NAME) {
String text = parser.getText();
if (parser.nextToken() != System.JSONToken.VALUE_NULL) {
if (text == 'count') {
count = parser.getIntegerValue();
} else if (text == 'next') {
next = parser.getText();
} else if (text == 'previous') {
previous = parser.readValueAs(Object.class);
} else if (text == 'results') {
results = arrayOfResults(parser);
} else {
System.debug(LoggingLevel.WARN, 'JSON2Apex consuming unrecognized property: '+text);
//consumeObject(parser);
}
}
}
}
}
private static List<Starship> arrayOfResults(System.JSONParser p) {
List<Starship> res = new List<Starship>();
if (p.getCurrentToken() == null) p.nextToken();
while (p.nextToken() != System.JSONToken.END_ARRAY) {
res.add(new Starship(p));
}
return res;
}
public static JSON2Apex parse(String json) {
System.JSONParser parser = System.JSON.createParser(json);
return new JSON2Apex(parser);
}
}